set_serializer.py
ofrak.service.serialization.serializers.set_serializer
FrozenSetSerializer (SerializerInterface)
Serialize and deserialize FrozenSet[X]
into PJSONType
.
Implementation: frozensets are serialized as lists.
obj_to_pjson(self, obj, type_hint)
Serialize the object to PJSON. Note that the generic self._service.to_pjson
can be used here.
Source code in ofrak/service/serialization/serializers/set_serializer.py
def obj_to_pjson(self, obj: FrozenSet[Any], type_hint: Any) -> List[PJSONType]:
args = get_args(type_hint)
return [self._service.to_pjson(item, args[0]) for item in obj]
pjson_to_obj(self, pjson_obj, type_hint)
Deserialize PJSON into the object. Note that the generic self._service.from_pjson
can be used here.
Source code in ofrak/service/serialization/serializers/set_serializer.py
def pjson_to_obj(self, pjson_obj: List[PJSONType], type_hint: Any) -> FrozenSet[Any]:
args = get_args(type_hint)
return frozenset(self._service.from_pjson(item, args[0]) for item in pjson_obj)
SetSerializer (SerializerInterface)
Serialize and deserialize Set[X]
into PJSONType
.
Implementation: sets are serialized as lists.
obj_to_pjson(self, obj, type_hint)
Serialize the object to PJSON. Note that the generic self._service.to_pjson
can be used here.
Source code in ofrak/service/serialization/serializers/set_serializer.py
def obj_to_pjson(self, obj: Set[Any], type_hint: Any) -> List[PJSONType]:
args = get_args(type_hint)
return [self._service.to_pjson(item, args[0]) for item in obj]
pjson_to_obj(self, pjson_obj, type_hint)
Deserialize PJSON into the object. Note that the generic self._service.from_pjson
can be used here.
Source code in ofrak/service/serialization/serializers/set_serializer.py
def pjson_to_obj(self, pjson_obj: List[PJSONType], type_hint: Any) -> Set[Any]:
args = get_args(type_hint)
return {self._service.from_pjson(item, args[0]) for item in pjson_obj}
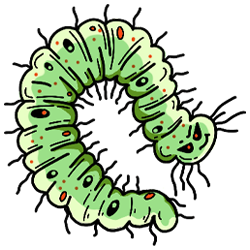