checksum.py
ofrak.core.checksum
Md5Analyzer (Analyzer)
Analyze binary data and add attributes with the MD5 checksum of the data.
analyze(self, resource, config=None)
async
Analyze a resource for to extract specific ResourceAttributes.
Users should not call this method directly; rather, they should run Resource.run or Resource.analyze.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
The resource that is being analyzed |
required |
config |
Optional config for analyzing. If an implementation provides a default, this default will always be used when config would otherwise be None. Note that a copy of the default config will be passed, so the default config values cannot be modified persistently by a component run. |
None |
Returns:
Type | Description |
---|---|
Md5Attributes |
The analysis results |
Source code in ofrak/core/checksum.py
async def analyze(self, resource: Resource, config=None) -> Md5Attributes:
data = await resource.get_data()
md5 = hashlib.md5()
md5.update(data)
return Md5Attributes(md5.hexdigest())
Md5Attributes (ResourceAttributes)
dataclass
Md5Attributes(checksum: str)
Sha256Analyzer (Analyzer)
Analyze binary data and add attributes with the SHA256 checksum of the data.
analyze(self, resource, config=None)
async
Analyze a resource for to extract specific ResourceAttributes.
Users should not call this method directly; rather, they should run Resource.run or Resource.analyze.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
The resource that is being analyzed |
required |
config |
Optional config for analyzing. If an implementation provides a default, this default will always be used when config would otherwise be None. Note that a copy of the default config will be passed, so the default config values cannot be modified persistently by a component run. |
None |
Returns:
Type | Description |
---|---|
Sha256Attributes |
The analysis results |
Source code in ofrak/core/checksum.py
async def analyze(self, resource: Resource, config=None) -> Sha256Attributes:
data = await resource.get_data()
sha256 = hashlib.sha256()
sha256.update(data)
return Sha256Attributes(sha256.hexdigest())
Sha256Attributes (ResourceAttributes)
dataclass
Sha256Attributes(checksum: str)
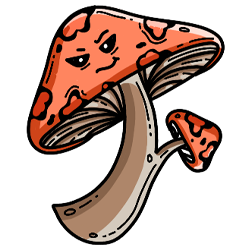