model.py
ofrak.core.pe.model
Pe (Program)
dataclass
PE file
get_sections(self)
async
Return the children PeSection
s.
Source code in ofrak/core/pe/model.py
async def get_sections(self) -> Iterable[PeSection]:
"""Return the children ``PeSection``s."""
return await self.resource.get_children_as_view(
PeSection,
ResourceFilter(
tags=(PeSection,),
),
)
get_optional_header(self)
async
Return the optional header of the PE file, or None if there isn't one.
Source code in ofrak/core/pe/model.py
async def get_optional_header(self) -> Optional[PeOptionalHeader]:
"""Return the optional header of the PE file, or None if there isn't one."""
try:
return await self.resource.get_only_child_as_view(
PeOptionalHeader,
ResourceFilter(
tags=(PeOptionalHeader,),
),
)
except NotFoundError:
return None
Pe32OptionalHeader (PeWinOptionalHeader)
dataclass
32 bit PE optional header with the base_of_data field.
Pe64POptionalHeader (PeWinOptionalHeader)
dataclass
64 bit PE optional header without the base_of_data field.
PeDataDirectory (ResourceView)
dataclass
PE data directory (image only)
PeFileHeader (ResourceView)
dataclass
PE file header, a.k.a. COFF file header
PeMsDosHeader (ResourceView)
dataclass
PE MS-DOS header
PeOptionalHeader (ResourceView)
dataclass
Base of PE optional header. Includes NT-specific attributes. This header class is valid for 64 Bit non-Windows PE files, however our pe_file package populates the additional Windows fields so this is for all intents and purposes an abstract class. More Info: https://learn.microsoft.com/en-us/windows/win32/debug/pe-format#optional-header-windows-specific-fields-image-only
Required for image files; object files don't have it.
PeOptionalHeaderMagic (Enum)
Magic values for the PE optional header
PeSection (PeSectionStructure, NamedProgramSection)
dataclass
PE section
PeSectionFlag (Enum)
Flags making up PeSectionHeader.m_characteristics
.
Refer to https://docs.microsoft.com/en-us/windows/win32/debug/pe-format#section-flags for documentation.
PeSectionHeader (PeSectionStructure)
dataclass
PE section header
name: str
property
readonly
The section name as a string, e.g. ".text" for b".text ".
get_body(self)
async
Get the PeSection associated with this section header.
Source code in ofrak/core/pe/model.py
async def get_body(self) -> PeSection:
"""Get the PeSection associated with this section header."""
return await self.resource.get_only_sibling_as_view(
PeSection,
ResourceFilter(
tags=(PeSection,),
attribute_filters=[
ResourceAttributeValueFilter(
PeSectionStructure.SectionIndex, self.section_index
)
],
),
)
PeSectionStructure (ResourceView)
dataclass
Superclass for both the section headers and the sections themselves, linking them via the section index.
PeWinOptionalHeader (PeOptionalHeader)
dataclass
PE Optional Header with all fields for Windows. These fields are not necessary, yet will be parsed by the pefile package regardless.
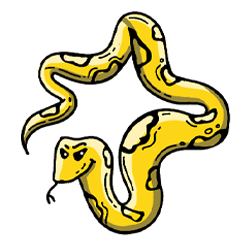