ref_analyzer.py
ofrak_ghidra.components.data.ref_analyzer
GhidraReferencedDataAnalyzer (ReferencedDataAnalyzer, OfrakGhidraMixin)
Analyzer to get all data references in the program
analyze(self, resource, config=None)
async
Analyze a resource for to extract specific ResourceAttributes.
Users should not call this method directly; rather, they should run Resource.run or Resource.analyze.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
The resource that is being analyzed |
required |
config |
Optional config for analyzing. If an implementation provides a default, this default will always be used when config would otherwise be None. Note that a copy of the default config will be passed, so the default config values cannot be modified persistently by a component run. |
None |
Returns:
Type | Description |
---|---|
Tuple[ofrak.core.data.ReferencedDataAttributes] |
The analysis results |
Source code in ofrak_ghidra/components/data/ref_analyzer.py
async def analyze(self, resource: Resource, config=None) -> Tuple[ReferencedDataAttributes]:
referenced_datas: List[int] = []
data_referenced_by_func: DefaultDict[int, List[int]] = defaultdict(list)
for data_ref_info in await self.get_data_refs_script.call_script(resource):
data_start = data_ref_info["data_address"]
referencing_funcs = data_ref_info["xrefs"]
data_idx = len(referenced_datas)
referenced_datas.append(data_start)
for func in referencing_funcs:
data_referenced_by_func[func].append(data_idx)
LOGGER.debug(f"adding reference to data 0x{data_start:x} for func 0x{func:x}")
referencing_funcs = list(data_referenced_by_func.keys())
edges = list()
for i, func in enumerate(referencing_funcs):
edges.extend([(i, j) for j in data_referenced_by_func[func]])
return (
ReferencedDataAttributes(
tuple(referencing_funcs),
tuple(referenced_datas),
tuple(edges),
),
)
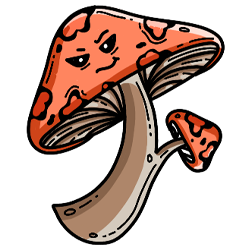