resource_view.py
ofrak.resource_view
ResourceView (ResourceViewInterface)
dataclass
An object representing a resource, or a future resource. May contain attributes and methods.
A class subclassing ResourceView
is a ResourceTag and
resources can be tagged with that tag. All ResourceView
s must be dataclasses. All fields of
the class will be represented by a ResourceAttributes
type, unless the field is private
(leading '_' in name).
A ResourceView
offers a synchronous view of all of the expected attributes of a resource with
a particular tag in one place.
Modifying the fields of a view will not modify the attributes of the underlying resource, nor
will modifying the attributes of the underlying resources automatically update the fields of an
existing view. Instead, the underlying resource should be explicitly modified and then a new
view should be created by calling resource.view_as(...)
again.
resource: Resource
property
writable
Getter to access the underlying resource.
Returns:
Type | Description |
---|---|
Resource |
the underlying resource |
Exceptions:
Type | Description |
---|---|
ValueError |
if there is no underlying resource |
get_attributes_instances(self)
Create instances of ResourceAttributes
for each of the composed attributes types of this
view. Each field of this view will end up in exactly one of these instances. Each
instance will have a different type.
Returns:
Type | Description |
---|---|
Dict[Type[~RA], ~RA] |
A dictionary mapping from subclasses of |
Source code in ofrak/resource_view.py
def get_attributes_instances(self) -> Dict[Type[RA], RA]:
"""
Create instances of `ResourceAttributes` for each of the composed attributes types of this
view. Each field of this view will end up in exactly one of these instances. Each
instance will have a different type.
:return: A dictionary mapping from subclasses of `ResourceAttributes` to a single instance
of each subclass.
"""
attrs_instances = dict()
for attrs_t in cast(Iterable[Type[RA]], type(self).composed_attributes_types):
attrs_fields_dict = {
field.name: getattr(self, field.name) for field in _fields(attrs_t) # type: ignore
}
attributes_instance = attrs_t(**attrs_fields_dict) # type: ignore
attrs_instances[attrs_t] = attributes_instance
return attrs_instances
set_deleted(self)
Mark that the underlying resource has been deleted.
Returns:
Type | Description |
---|---|
Source code in ofrak/resource_view.py
def set_deleted(self):
self._resource = None
self._deleted = True
tag_specificity(cls)
classmethod
Override ResourceTag.tag_specificity to indicate this is not a valid tag, but its subclasses will be.
Returns:
Type | Description |
---|---|
int |
Source code in ofrak/resource_view.py
@classmethod
@functools.lru_cache(None)
def tag_specificity(cls) -> int:
"""
Override [ResourceTag.tag_specificity][ofrak.model.tag_model.ResourceTag.tag_specificity]
to indicate this is not a valid tag, but its subclasses will be.
:return:
"""
if cls is ResourceView or cls is ResourceViewInterface:
return -1
else:
return ResourceTag.tag_specificity(cls)
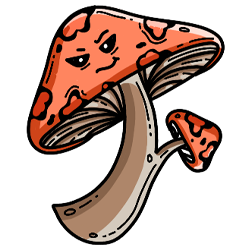