stashed_pjson.py
ofrak.service.serialization.stashed_pjson
StashedPJSONSerializationService (SerializationServiceInterface)
Serialization service producing a more compact encoding than PJSONSerializationService
, at the expense of time
performance, by mapping each string in the PJSON to a shorter one, and including the mapping at the beginning of
the serialized form.
_convert(self, pjson, string_mapping)
private
Perform the string replacement according to string_mapping
.
This method is used for both encoding and decoding, with opposite string_mapping
dictionaries.
Source code in ofrak/service/serialization/stashed_pjson.py
def _convert(
self,
pjson: PJSONType,
string_mapping: Dict[str, str],
) -> PJSONType:
"""
Perform the string replacement according to `string_mapping`.
This method is used for both encoding and decoding, with opposite `string_mapping` dictionaries.
"""
if isinstance(pjson, str):
return string_mapping[pjson]
elif isinstance(pjson, list):
return [self._convert(item, string_mapping) for item in pjson]
elif isinstance(pjson, tuple):
return tuple(self._convert(item, string_mapping) for item in pjson)
elif isinstance(pjson, dict):
return {
cast(str, self._convert(key, string_mapping)): self._convert(value, string_mapping)
for key, value in pjson.items()
}
else:
return pjson
_short_string_generator()
private
Generator of short JSON-compatible strings of increasing size.
Source code in ofrak/service/serialization/stashed_pjson.py
def _short_string_generator():
"""Generator of short JSON-compatible strings of increasing size."""
disallowed_characters = [0x22, 0x5C, 0x2F, 0x62, 0x66, 0x6E, 0x72, 0x74, 0x75]
alphabet = [chr(i) for i in range(0x20, 0x7E) if i not in disallowed_characters]
size = 1
while True:
for letters in product(*([alphabet] * size)):
yield "".join(letters)
size += 1
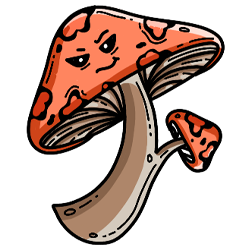