model.py
ofrak.core.elf.model
Elf (Program)
dataclass
An Executable and Linking Format (ELF) file.
See https://man7.org/linux/man-pages/man5/elf.5.html for details.
ElfBasicHeader (ResourceView)
dataclass
See "e_ident" in https://man7.org/linux/man-pages/man5/elf.5.html for details.
ElfDynamicEntry (ResourceView)
dataclass
ElfDynamicEntry describes a .dynamic table entry.
https://docs.oracle.com/cd/E23824_01/html/819-0690/chapter6-42444.html
Attributes:
Name | Type | Description |
---|---|---|
d_tag |
int |
one of ElfDynamicTableTag |
d_un |
int |
malleable word size value that changes meaning depending on d_tag |
ElfDynamicSection (ElfSection)
The .dynamic ELF Section that appears in dynamically linked ELFs.
ElfDynamicTableTag (Enum)
An enumeration.
ElfHeader (ResourceView)
dataclass
See "ELF header (Ehdr)" in https://man7.org/linux/man-pages/man5/elf.5.html for details.
ElfMachine (Enum)
An enumeration.
ElfPointerArraySection (ElfSection)
dataclass
An ELF Section that can be interpreted as an array of pointers.
TODO: Except in the case of .init_array and .fini_array, this tag must be added by hand as overlapping with other tags, like CodeRegion results in Data Node inconsistencies and errors.
ElfProgramHeader (ElfSegmentStructure)
dataclass
See "Program header (Phdr)" in https://man7.org/linux/man-pages/man5/elf.5.html for details.
get_memory_permissions(self)
Get the MemoryPermission for the ElfProgramHeader.
Source code in ofrak/core/elf/model.py
def get_memory_permissions(self) -> MemoryPermissions:
"""
Get the MemoryPermission for the ElfProgramHeader.
"""
return MemoryPermissions(self.p_flags & 0b111)
ElfProgramHeaderType (Enum)
ElfRelaEntry (ResourceView)
dataclass
ElfRelaEntry describes relocation information within the program. Located in .rela.* sections.
Attributes:
Name | Type | Description |
---|---|---|
r_offset |
int |
vm offset information for each relocation entry |
r_info |
int |
Describes the type of relocation and sometimes the symbol related to the relocation |
r_addend |
int |
Describes the VM offset for each relocation itself |
ElfRelaInfo (Enum)
An Enum for r_info in ElfRela
Implemented in each respective arch model.py
ElfRelaSection (ElfSection)
An ELF .rela.* section containing structs of type Elf{32, 64}_Rela
ElfSection (ElfSectionStructure, NamedProgramSection)
dataclass
An ELF Section.
ElfSectionFlag (Enum)
An enumeration.
ElfSectionHeader (ElfSectionStructure)
dataclass
See "Section header (Shdr)" in https://man7.org/linux/man-pages/man5/elf.5.html for details.
ElfSectionNameStringSection (ElfStringSection)
A section with the STRTAB flag and named ".shstrtab". There should be at most one of these per ELF, and it contains only strings for the names of sections. Section headers' sh_name field is an index within this .shstrtab section.
ElfSectionStructure (ResourceView)
dataclass
ElfSectionStructure(section_index: int)
ElfSectionType (Enum)
An enumeration.
ElfSegment (ElfSegmentStructure, ProgramSegment)
dataclass
An ELF Segment.
ElfSegmentStructure (ResourceView)
dataclass
ElfSegmentStructure(segment_index: int)
ElfStringSection (ElfSectionStructure)
A section with the STRTAB flag. There may be several of these in an ELF.
ElfSymbol (ElfSymbolStructure)
dataclass
See "String and symbol tables" in https://man7.org/linux/man-pages/man5/elf.5.html for details.
ElfSymbolBinding (Enum)
An enumeration.
ElfSymbolSection (ElfSection)
An ELF section containing structures of type Elf{32, 64}_Sym
ElfSymbolStructure (ResourceView)
dataclass
ElfSymbolStructure(symbol_index: int)
ElfSymbolType (Enum)
An enumeration.
ElfSymbolVisibility (Enum)
An enumeration.
ElfType (Enum)
An enumeration.
ElfVirtualAddress (ResourceView)
dataclass
Wrapper for a virtual address
Attributes:
Name | Type | Description |
---|---|---|
value |
int |
an address |
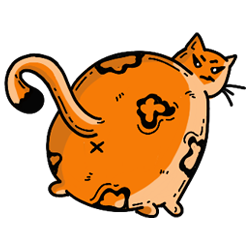