resource_model_serializer.py
ofrak.service.serialization.serializers.resource_model_serializer
ResourceModelSerializer (SerializerInterface)
Serialize and deserialize ResourceModel
into PJSONType
.
There are two issues with ResourceModel: 1) the type hint of ResourceModel.attributes = Dict[Type[RA], RA] with RA = TypeVar("RA", bound="ResourceAttributes") This is used to refer to ResourceAttributes in the type hints without creating circular imports. But the serializer needs to know the real class ResourceAttributes, not just its name. 2) the type hint of ResourceModel.tags is SortedSet[ResourceTag], but the generic serializer doesn't know how to handle SortedSet (to do it right, we would also need to serialize the sorting function, not just the sequence). But tags can be given to ResourceModel.init as simply any Sequence, so we use that type hint instead.
obj_to_pjson(self, obj, _type_hint)
Serialize the object to PJSON. Note that the generic self._service.to_pjson
can be used here.
Source code in ofrak/service/serialization/serializers/resource_model_serializer.py
def obj_to_pjson(self, obj: Any, _type_hint: Any) -> Dict[str, PJSONType]:
result = {}
for attr_name, type_hint in self.usable_type_hints.items():
result[attr_name] = self._service.to_pjson(getattr(obj, attr_name), type_hint)
# Manually add captions to the generated dictionary since they are a read-only property,
# and they should only be serialized, never deserialized and passed to an object's __init__
result["caption"] = obj.caption
return result
pjson_to_obj(self, pjson_obj, _type_hint)
Deserialize PJSON into the object. Note that the generic self._service.from_pjson
can be used here.
Source code in ofrak/service/serialization/serializers/resource_model_serializer.py
def pjson_to_obj(self, pjson_obj: Dict[str, PJSONType], _type_hint: Any) -> Any:
deserialized_attrs = {
attr_name: self._service.from_pjson(pjson_obj[attr_name], type_hint)
for attr_name, type_hint in self.usable_type_hints.items()
}
return ResourceModel(**deserialized_attrs)
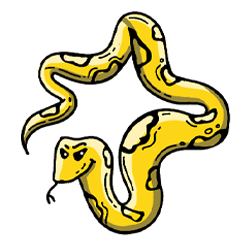