binary.py
ofrak.core.binary
BinaryExtendConfig (ComponentConfig)
dataclass
Config for the BinaryExtendModifier.
Attributes:
Name | Type | Description |
---|---|---|
content |
bytes |
the extended bytes. |
BinaryExtendModifier (Modifier)
Extend a binary with new data.
modify(self, resource, config)
async
Modify the given resource.
Users should not call this method directly; rather, they should run Resource.run.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
required | |
config |
BinaryExtendConfig |
Optional config for modification. If an implementation provides a default, this default will always be used when config would otherwise be None. Note that a copy of the default config will be passed, so the default config values cannot be modified persistently by a component run. |
required |
Source code in ofrak/core/binary.py
async def modify(self, resource: Resource, config: BinaryExtendConfig):
if len(config.content) == 0:
raise ValueError("Content of the extended space not provided")
data = await resource.get_data()
data += config.content
resource.queue_patch(Range(0, await resource.get_data_length()), data)
BinaryPatchConfig (ComponentConfig)
dataclass
Config for the BinaryPatchModifier.
Attributes:
Name | Type | Description |
---|---|---|
offset |
int |
physical offset from beginning of resource where the patch should be applied |
patch_bytes |
bytes |
the raw bytes to patch |
BinaryPatchModifier (Modifier)
Patch a binary at the target offset with the given patch bytes.
modify(self, resource, config)
async
Patch the resource at the target offset with the given patch bytes.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
the resource to patch |
required |
config |
BinaryPatchConfig |
contains the offset at which to patch and the patch bytes to apply |
required |
Exceptions:
Type | Description |
---|---|
ModifierError |
if the binary patch overflows the original size of the resource |
Source code in ofrak/core/binary.py
async def modify(self, resource: Resource, config: BinaryPatchConfig) -> None:
"""
Patch the resource at the target offset with the given patch bytes.
:param resource: the resource to patch
:param config: contains the offset at which to patch and the patch bytes to apply
:raises ModifierError: if the binary patch overflows the original size of the resource
"""
resource_size = await resource.get_data_length()
if len(config.patch_bytes) > resource_size - config.offset:
raise ModifierError(
f"The binary patch, {config}, overflows the original size of the resource "
f"{resource.get_id().hex()}."
)
resource.queue_patch(config.get_range(), config.patch_bytes)
return
GenericBinary (ResourceView)
A generic binary blob.
GenericText (GenericBinary)
A binary that consists of lines of text.
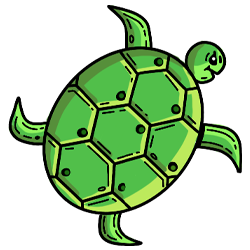