assembler_service_i.py
ofrak.service.assembler.assembler_service_i
Assembler services used to assemble and disassemble code.
AssemblerServiceInterface (AbstractOfrakService)
An interface for assembler services.
__metaclass__ (type)
Metaclass for defining Abstract Base Classes (ABCs).
Use this metaclass to create an ABC. An ABC can be subclassed directly, and then acts as a mix-in class. You can also register unrelated concrete classes (even built-in classes) and unrelated ABCs as 'virtual subclasses' -- these and their descendants will be considered subclasses of the registering ABC by the built-in issubclass() function, but the registering ABC won't show up in their MRO (Method Resolution Order) nor will method implementations defined by the registering ABC be callable (not even via super()).
__new__(mcls, name, bases, namespace, **kwargs)
special
staticmethod
Create and return a new object. See help(type) for accurate signature.
Source code in ofrak/service/assembler/assembler_service_i.py
def __new__(mcls, name, bases, namespace, **kwargs):
cls = super().__new__(mcls, name, bases, namespace, **kwargs)
_abc_init(cls)
return cls
register(cls, subclass)
Register a virtual subclass of an ABC.
Returns the subclass, to allow usage as a class decorator.
Source code in ofrak/service/assembler/assembler_service_i.py
def register(cls, subclass):
"""Register a virtual subclass of an ABC.
Returns the subclass, to allow usage as a class decorator.
"""
return _abc_register(cls, subclass)
__instancecheck__(cls, instance)
special
Override for isinstance(instance, cls).
Source code in ofrak/service/assembler/assembler_service_i.py
def __instancecheck__(cls, instance):
"""Override for isinstance(instance, cls)."""
return _abc_instancecheck(cls, instance)
__subclasscheck__(cls, subclass)
special
Override for issubclass(subclass, cls).
Source code in ofrak/service/assembler/assembler_service_i.py
def __subclasscheck__(cls, subclass):
"""Override for issubclass(subclass, cls)."""
return _abc_subclasscheck(cls, subclass)
_dump_registry(cls, file=None)
private
Debug helper to print the ABC registry.
Source code in ofrak/service/assembler/assembler_service_i.py
def _dump_registry(cls, file=None):
"""Debug helper to print the ABC registry."""
print(f"Class: {cls.__module__}.{cls.__qualname__}", file=file)
print(f"Inv. counter: {get_cache_token()}", file=file)
(_abc_registry, _abc_cache, _abc_negative_cache,
_abc_negative_cache_version) = _get_dump(cls)
print(f"_abc_registry: {_abc_registry!r}", file=file)
print(f"_abc_cache: {_abc_cache!r}", file=file)
print(f"_abc_negative_cache: {_abc_negative_cache!r}", file=file)
print(f"_abc_negative_cache_version: {_abc_negative_cache_version!r}",
file=file)
_abc_registry_clear(cls)
private
Clear the registry (for debugging or testing).
Source code in ofrak/service/assembler/assembler_service_i.py
def _abc_registry_clear(cls):
"""Clear the registry (for debugging or testing)."""
_reset_registry(cls)
_abc_caches_clear(cls)
private
Clear the caches (for debugging or testing).
Source code in ofrak/service/assembler/assembler_service_i.py
def _abc_caches_clear(cls):
"""Clear the caches (for debugging or testing)."""
_reset_caches(cls)
assemble(self, assembly, vm_addr, program_attributes, mode=<InstructionSetMode.NONE: 0>)
async
Assemble the given assembly code.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
assembly |
str |
The assembly to assemble |
required |
vm_addr |
int |
The virtual address at which the assembly should be assembled. |
required |
program_attributes |
ProgramAttributes |
The processor targeted by the assembly |
required |
mode |
InstructionSetMode |
The mode of the processor for the assembly |
<InstructionSetMode.NONE: 0> |
Returns:
Type | Description |
---|---|
bytes |
The assembled machine code |
Source code in ofrak/service/assembler/assembler_service_i.py
@abstractmethod
async def assemble(
self,
assembly: str,
vm_addr: int,
program_attributes: ProgramAttributes,
mode: InstructionSetMode = InstructionSetMode.NONE,
) -> bytes:
"""
Assemble the given assembly code.
:param str assembly: The assembly to assemble
:param int vm_addr: The virtual address at which the assembly should be assembled.
:param ProgramAttributes program_attributes: The processor targeted by the assembly
:param InstructionSetMode mode: The mode of the processor for the assembly
:return: The assembled machine code
"""
raise NotImplementedError
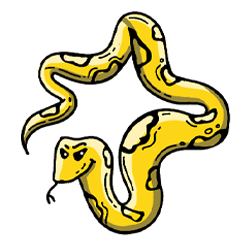