component_model.py
ofrak.model.component_model
ClientComponentContext (ComponentContext)
__init__(self)
special
Initialize self. See help(type(self)) for accurate signature.
Source code in ofrak/model/component_model.py
def __init__(self):
super().__init__(CLIENT_COMPONENT_ID, CLIENT_COMPONENT_VERSION)
ComponentConfig
dataclass
Base class for all components' configs. All subclasses should also be dataclasses.
ComponentContext
dataclass
ComponentContext(component_id: bytes, component_version: int, access_trackers: Dict[bytes, ofrak.model.component_model.ComponentResourceAccessTracker] =
ComponentExternalTool
dataclass
An external tool or utility (like zip
or squashfs
) a component depends on. Includes some
basic information on installation, either via package manager or bespoke process.
Part of this class's responsibility is to check if the tool is installed. Most tools are
simple command-line utilities whose installation can be check by running:
<tool> <install_check_arg>
For dependencies which do NOT follow this pattern, subclass ComponentExternalTool and redefine
the is_tool_installed
method to perform the check.
Attributes:
Name | Type | Description |
---|---|---|
tool |
str |
Name of the command-line tool that will be run |
tool_homepage |
str |
Link to homepage of the tool, with install instructions etc. |
install_check_arg |
str |
Argument to pass to the tool to check if it can be found and run on the host, typically something like "--help" |
apt_package |
Optional[str] |
An |
brew_package |
Optional[str] |
An |
is_tool_installed(self)
async
Check if a tool is installed by running it with the install_check_arg
.
This method runs <tool> <install_check_arg>
.
Returns:
Type | Description |
---|---|
bool |
True if the |
Source code in ofrak/model/component_model.py
async def is_tool_installed(self) -> bool:
"""
Check if a tool is installed by running it with the `install_check_arg`.
This method runs `<tool> <install_check_arg>`.
:return: True if the `tool` command returned zero, False if `tool` could not be found or
returned non-zero exit code.
"""
try:
cmd = [
self.tool,
self.install_check_arg,
]
proc = await asyncio.create_subprocess_exec(
*cmd,
stdout=asyncio.subprocess.DEVNULL,
stderr=asyncio.subprocess.DEVNULL,
)
returncode = await proc.wait()
except FileNotFoundError:
return False
return 0 == returncode
ComponentResourceAccessTracker
dataclass
ComponentResourceAccessTracker(data_accessed: Set[ofrak_type.range.Range] =
ComponentResourceModificationTracker
dataclass
ComponentResourceModificationTracker(data_patches: List[ofrak.model.data_model.DataPatch] =
ComponentRunResult
dataclass
Dataclass created after one or more components complete, holding high-level information about what resources were affected by a component or components
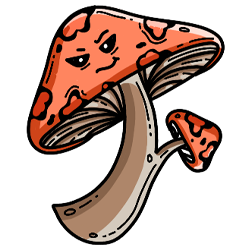