id_service_i.py
ofrak.service.id_service_i
IDServiceInterface (AbstractOfrakService, ABC)
The IDServiceInterface
is an interface for a service that generates unique IDs.
This interface is intended to be a singleton used to create IDs locally; if more than one instance of this service is going to be instantiated, then this service must be stateless and not rely on any internal state.
generate_id(self)
Generate a unique ID.
This method guarantees that no two IDs it returns will be identical for the lifetime of
the instantiated IDServiceInterface
.
Returns:
Type | Description |
---|---|
bytes |
The unique ID that was generated. |
Source code in ofrak/service/id_service_i.py
@abstractmethod
def generate_id(self) -> bytes:
"""
Generate a unique ID.
This method guarantees that no two IDs it returns will be identical for the lifetime of
the instantiated `IDServiceInterface`.
:return: The unique ID that was generated.
"""
raise NotImplementedError()
generate_id_from_base(base_id, key)
staticmethod
Generate an ID based on a base ID and key.
This method returns the same ID each time it is called with the exact same parameters. It should therefore be used with caution: the callee needs to know that the parameters passed to this method are unique.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
base_id |
bytes |
An ID used to derive the generated ID. This ID can be assumed to have been generated by the |
required |
key |
str |
A key used to guarantee that the generated ID is unique. The callee is responsible for ensuring that this key is unique. |
required |
Returns:
Type | Description |
---|---|
bytes |
The ID that was generated. |
Source code in ofrak/service/id_service_i.py
@staticmethod
@abstractmethod
def generate_id_from_base(base_id: bytes, key: str) -> bytes:
"""
Generate an ID based on a base ID and key.
This method returns the same ID each time it is called with the exact same parameters. It
should therefore be used with caution: the callee needs to know that the parameters
passed to this method are unique.
:param bytes base_id: An ID used to derive the generated ID. This ID can be assumed to have
been generated by the `generate_id` method.
:param str key: A key used to guarantee that the generated ID is unique. The callee is
responsible for ensuring that this key is unique.
:return: The ID that was generated.
"""
raise NotImplementedError()
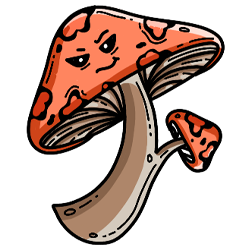