dict_serializer.py
ofrak.service.serialization.serializers.dict_serializer
DictSerializer (SerializerInterface)
Serialize and deserialize Dict[X, Y]
into PJSONType
.
Implementation: a list of tuples (key, value), because the serialized keys may not be hashable (e.g. if they're themselves serialized as a dictionary), despite the real keys being hashable.
obj_to_pjson(self, obj, type_hint)
Serialize the object to PJSON. Note that the generic self._service.to_pjson
can be used here.
Source code in ofrak/service/serialization/serializers/dict_serializer.py
def obj_to_pjson(self, obj: Dict[Any, Any], type_hint: Any) -> SerializedDictType:
key_type, value_type = get_args(type_hint)
return [
(self._service.to_pjson(key, key_type), self._service.to_pjson(value, value_type))
for key, value in obj.items()
]
pjson_to_obj(self, pjson_obj, type_hint)
Deserialize PJSON into the object. Note that the generic self._service.from_pjson
can be used here.
Source code in ofrak/service/serialization/serializers/dict_serializer.py
def pjson_to_obj(self, pjson_obj: SerializedDictType, type_hint: Any) -> Dict[Any, Any]:
key_type, value_type = get_args(type_hint)
return {
self._service.from_pjson(key, key_type): self._service.from_pjson(value, value_type)
for key, value in pjson_obj
}
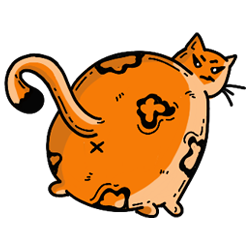