gzip.py
ofrak.core.gzip
GzipData (GenericBinary)
A gzip binary blob.
GzipPacker (Packer)
Pack data into a compressed gzip file.
pack(self, resource, config=None)
async
Pack the given resource.
Users should not call this method directly; rather, they should run Resource.run or Resource.pack.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
required | |
config |
Optional config for packing. If an implementation provides a default, this default will always be used when config would otherwise be None. Note that a copy of the default config will be passed, so the default config values cannot be modified persistently by a component run. |
None |
Source code in ofrak/core/gzip.py
async def pack(self, resource: Resource, config=None):
gzip_view = await resource.view_as(GzipData)
gzip_child_r = await gzip_view.get_file()
data = await gzip_child_r.get_data()
if len(data) >= 1024 * 1024 and await PIGZInstalled.is_pigz_installed():
packed_data = await self.pack_with_pigz(data)
else:
packed_data = await self.pack_with_zlib_module(data)
original_gzip_size = await gzip_view.resource.get_data_length()
resource.queue_patch(Range(0, original_gzip_size), data=packed_data)
GzipUnpacker (Unpacker)
Unpack (decompress) a gzip file.
unpack(self, resource, config=None)
async
Unpack the given resource.
Users should not call this method directly; rather, they should run Resource.run or Resource.unpack.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
The resource that is being unpacked |
required |
config |
Optional config for unpacking. If an implementation provides a default, this default will always be used when config would otherwise be None. Note that a copy of the default config will be passed, so the default config values cannot be modified persistently by a component run. |
None |
Source code in ofrak/core/gzip.py
async def unpack(self, resource: Resource, config=None):
data = await resource.get_data()
unpacked_data = await self.unpack_with_zlib_module(data)
return await resource.create_child(tags=(GenericBinary,), data=unpacked_data)
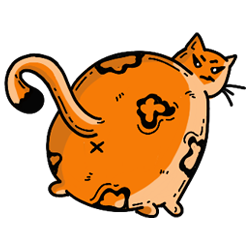