strings_analyzer.py
ofrak.core.strings_analyzer
StringsAnalyzer (Analyzer)
analyze(self, resource, config=None)
async
Analyze a resource for to extract specific ResourceAttributes.
Users should not call this method directly; rather, they should run Resource.run or Resource.analyze.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
The resource that is being analyzed |
required |
config |
Optional[ofrak.core.strings_analyzer.StringsAnalyzerConfig] |
Optional config for analyzing. If an implementation provides a default, this default will always be used when config would otherwise be None. Note that a copy of the default config will be passed, so the default config values cannot be modified persistently by a component run. |
None |
Returns:
Type | Description |
---|---|
StringsAttributes |
The analysis results |
Source code in ofrak/core/strings_analyzer.py
async def analyze(
self, resource: Resource, config: Optional[StringsAnalyzerConfig] = None
) -> StringsAttributes:
if config is None:
config = StringsAnalyzerConfig()
strings = dict()
async with resource.temp_to_disk() as temp_path:
proc = await asyncio.subprocess.create_subprocess_exec(
"strings",
"-t",
"d",
f"-{config.min_length}",
temp_path,
stdout=asyncio.subprocess.PIPE,
)
line = await proc.stdout.readline() # type: ignore
while line:
line = line.decode("ascii").strip()
try:
offset, string = line.split(" ", maxsplit=1)
except ValueError as e:
# String consisted entirely of whitespace
line = await proc.stdout.readline() # type: ignore
continue
strings[int(offset)] = string
line = await proc.stdout.readline() # type: ignore
await proc.wait()
return StringsAttributes(strings)
StringsAnalyzerConfig (ComponentConfig)
dataclass
StringsAnalyzerConfig(min_length: int = 8)
StringsAttributes (ResourceAttributes)
dataclass
StringsAttributes(strings: Dict[int, str])
_StringsToolDependency (ComponentExternalTool)
private
__init__(self)
special
Initialize self. See help(type(self)) for accurate signature.
Source code in ofrak/core/strings_analyzer.py
def __init__(self):
super().__init__(
"strings",
"https://linux.die.net/man/1/strings",
"--help",
apt_package="binutils",
brew_package="binutils",
)
is_tool_installed(self)
async
Check if a tool is installed by running it with the install_check_arg
.
This method runs <tool> <install_check_arg>
.
Returns:
Type | Description |
---|---|
bool |
True if the |
Source code in ofrak/core/strings_analyzer.py
async def is_tool_installed(self) -> bool:
try:
cmd = [
self.tool,
self.install_check_arg,
]
proc = await asyncio.create_subprocess_exec(
*cmd,
stdout=asyncio.subprocess.DEVNULL,
stderr=asyncio.subprocess.DEVNULL,
)
# ignore returncode because "strings --help" on Mac has returncode 1
await proc.wait()
except FileNotFoundError:
return False
return True
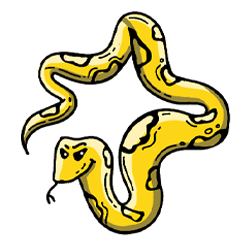