model.py
ofrak_patch_maker.model
These classes must only ever contain "immutable" data.
We never want to worry about the state of these objects at any point during a patch injection.
AssembledObject
dataclass
The result of any compile
or assemble
step
Attributes:
Name | Type | Description |
---|---|---|
path |
str |
.o file path |
file_format |
BinFileType |
.elf, .coff, etc. |
segment_map |
Mapping[str, ofrak_patch_maker.toolchain.model.Segment] |
e.g. |
strong_symbols |
Mapping[str, Tuple[int, ofrak_type.symbol_type.LinkableSymbolType]] |
|
unresolved_symbols |
Mapping[str, Tuple[int, ofrak_type.symbol_type.LinkableSymbolType]] |
{symbol name: (address, symbol type)} |
bss_size_required |
Optional[int] |
DEPRECATED |
BOM
dataclass
"BOM" stands for Batched Objects and Metadata
Keeping this class frozen ensures we have a hash for every instance.
Instances of this class should never be declared outside of PatchMaker.
Attributes:
Name | Type | Description |
---|---|---|
name |
str |
a name |
object_map |
Mapping[str, ofrak_patch_maker.model.AssembledObject] |
{source file path: AssembledObject} |
unresolved_symbols |
Set[str] |
symbols used but undefined within the BOM source files |
bss_size_required |
Optional[int] |
DEPRECATED |
entry_point_symbol |
Optional[str] |
symbol of the patch entrypoint, when relevant |
FEM
dataclass
"FEM" stands for Final Executable and Metadata
Keeping this class frozen ensures we have a hash for every instance
Instances of this class should never be declared outside of PatchMaker.
Attributes:
Name | Type | Description |
---|---|---|
name |
str |
|
executable |
LinkedExecutable |
LinkedExecutable
dataclass
The result of any link
step.
Attributes:
Name | Type | Description |
---|---|---|
path |
str |
executable file path |
file_format |
BinFileType |
.elf, .coff, etc. |
segments |
Tuple[ofrak_patch_maker.toolchain.model.Segment, ...] |
the segments contained in this executable |
symbols |
Mapping[str, Tuple[int, ofrak_type.symbol_type.LinkableSymbolType]] |
the global function and data symbol mapping information for this executable |
relocatable |
bool |
TODO: whether or not the executable is relocatable |
PatchRegionConfig
dataclass
PatchRegionConfig is the dataclass required to generate an .ld script. That describes a set of code and data patches.
For every available object file, new code and data Segments are populated with the target VM addresses/sizes within the client firmware.
PatchMaker uses this information to generate memory regions and the sections that will go into those memory regions.
Attributes:
Name | Type | Description |
---|---|---|
patch_name |
str |
a name |
segments |
Mapping[str, Tuple[ofrak_patch_maker.toolchain.model.Segment, ...]] |
{object file path: Tuple of Segments} |
SourceFileType (Enum)
An enumeration.
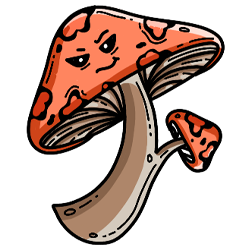