Registering Identifier Patterns
When writing unpackers, OFRAK Contributors can leverage the MagicMimeIdentifier
and MagicDescriptionIdentifier
by registering mappings between resource tags and mime or description patterns. Doing so will ensure that Resource.unpack
automatically calls their custom unpacker.
For example, consider the following magic description identification registration in the file containing a UImageUnpacker
:
MagicDescriptionIdentifier.register(UImage, lambda s: s.startswith("u-boot legacy uImage"))
This line ensures that the MagicDescriptionIdentifier
adds a UImage
tag to resources matching that description pattern. As a result, any unpackers targeting a UImage
will automatically run when Resource.unpack
is run.
Handling External Dependencies
If the Identifier makes use of tools that are not packaged in modules installable via pip
from
PyPI (commonly command-line tools), these dependencies must be explicitly declared as part of the
identifier's class declaration. See the Components Using External Tools doc
for information on how to do that.
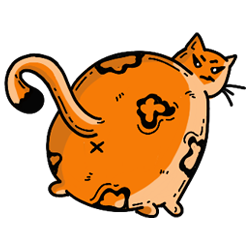