magic.py
ofrak.core.magic
Magic (ResourceAttributes)
dataclass
Magic(mime: str, descriptor: str)
MagicAnalyzer (Analyzer)
Analyze a binary blob to extract its mimetype and magic description.
analyze(self, resource, config=None)
async
Analyze a resource for to extract specific ResourceAttributes.
Users should not call this method directly; rather, they should run Resource.run or Resource.analyze.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
The resource that is being analyzed |
required |
config |
Optional config for analyzing. If an implementation provides a default, this default will always be used when config would otherwise be None. Note that a copy of the default config will be passed, so the default config values cannot be modified persistently by a component run. |
None |
Returns:
Type | Description |
---|---|
Magic |
The analysis results |
Source code in ofrak/core/magic.py
async def analyze(self, resource: Resource, config=None) -> Magic:
data = await resource.get_data()
if not MAGIC_INSTALLED:
raise ComponentMissingDependencyError(self, LIBMAGIC_DEP)
else:
magic_mime = magic.from_buffer(data, mime=True)
magic_description = magic.from_buffer(data)
return Magic(magic_mime, magic_description)
MagicDescriptionIdentifier (Identifier)
Identify and add the appropriate tag for a given resource based on its mime description.
identify(self, resource, config)
async
Perform identification on the given resource.
Users should not call this method directly; rather, they should run Resource.identify.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
required | |
config |
Optional config for identifying. If an implementation provides a default, this default will always be used when config would otherwise be None. Note that a copy of the default config will be passed, so the default config values cannot be modified persistently by a component run. |
required |
Source code in ofrak/core/magic.py
async def identify(self, resource: Resource, config):
_magic = await resource.analyze(Magic)
magic_description = _magic.descriptor
for matcher, resource_type in self._matchers.items():
if matcher(magic_description):
resource.add_tag(resource_type)
register(resource, matcher)
classmethod
Register a virtual subclass of an ABC.
Returns the subclass, to allow usage as a class decorator.
Source code in ofrak/core/magic.py
@classmethod
def register(cls, resource: ResourceTag, matcher: Callable):
if matcher in cls._matchers:
raise AlreadyExistError("Registering already-registered matcher")
cls._matchers[matcher] = resource
MagicMimeIdentifier (Identifier)
Identify and add the appropriate tag for a given resource based on its mimetype.
identify(self, resource, config=None)
async
Perform identification on the given resource.
Users should not call this method directly; rather, they should run Resource.identify.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
required | |
config |
Optional config for identifying. If an implementation provides a default, this default will always be used when config would otherwise be None. Note that a copy of the default config will be passed, so the default config values cannot be modified persistently by a component run. |
None |
Source code in ofrak/core/magic.py
async def identify(self, resource: Resource, config=None):
_magic = await resource.analyze(Magic)
magic_mime = _magic.mime
tag = MagicMimeIdentifier._tags_by_mime.get(magic_mime)
if tag is not None:
resource.add_tag(tag)
register(resource, mime_types)
classmethod
Register a virtual subclass of an ABC.
Returns the subclass, to allow usage as a class decorator.
Source code in ofrak/core/magic.py
@classmethod
def register(cls, resource: ResourceTag, mime_types: Union[Iterable[str], str]):
if isinstance(mime_types, str):
mime_types = [mime_types]
for mime_type in mime_types:
if mime_type in cls._tags_by_mime:
raise AlreadyExistError(f"Registering already-registered mime type: {mime_type}")
cls._tags_by_mime[mime_type] = resource
_LibmagicDependency (ComponentExternalTool)
private
__init__(self)
special
Initialize self. See help(type(self)) for accurate signature.
Source code in ofrak/core/magic.py
def __init__(self):
super().__init__(
"libmagic",
"https://www.darwinsys.com/file/",
install_check_arg="",
apt_package="libmagic1",
brew_package="libmagic",
)
try:
import magic as _magic
_LibmagicDependency._magic = _magic
except ImportError:
_LibmagicDependency._magic = None
is_tool_installed(self)
async
Check if a tool is installed by running it with the install_check_arg
.
This method runs <tool> <install_check_arg>
.
Returns:
Type | Description |
---|---|
bool |
True if the |
Source code in ofrak/core/magic.py
async def is_tool_installed(self) -> bool:
return MAGIC_INSTALLED
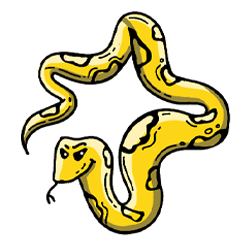