analyzer.py
ofrak.core.elf.analyzer
ElfBasicHeaderAttributesAnalyzer (Analyzer)
Deserialize the ElfBasicHeader, which contains the first 7 fields of the ELF header. These fields are all endianness- & word-size-agnostic, and in fact define the endianness and word size for the remainder of the header.
The remaining fields are deserialized as part of the ElfHeader. See "ELF header (Ehdr)" in https://man7.org/linux/man-pages/man5/elf.5.html for details.
analyze(self, resource, config=None)
async
Analyze a resource for to extract specific ResourceAttributes.
Users should not call this method directly; rather, they should run Resource.run or Resource.analyze.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
The resource that is being analyzed |
required |
config |
Optional config for analyzing. If an implementation provides a default, this default will always be used when config would otherwise be None. Note that a copy of the default config will be passed, so the default config values cannot be modified persistently by a component run. |
None |
Returns:
Type | Description |
---|---|
ElfBasicHeader |
The analysis results |
Source code in ofrak/core/elf/analyzer.py
async def analyze(self, resource: Resource, config=None) -> ElfBasicHeader:
tmp = await resource.get_data()
deserializer = BinaryDeserializer(io.BytesIO(tmp))
(
ei_magic,
ei_class,
ei_data,
ei_version,
ei_osabi,
ei_abiversion,
ei_pad,
) = deserializer.unpack_multiple("4sBBBBB7s")
assert ei_magic == b"\x7fELF"
return ElfBasicHeader(
ei_magic, ei_class, ei_data, ei_version, ei_osabi, ei_abiversion, ei_pad
)
ElfDynamicSectionAnalyzer (Analyzer)
If an object file participates in dynamic linking, its program header table will have an element of type PT_DYNAMIC. This segment contains the .dynamic section.
Descriptions of the Dynamic Table entries may be found herein: https://docs.oracle.com/cd/E19683-01/817-3677/chapter6-42444/index.html
analyze(self, resource, config=None)
async
Analyze a resource for to extract specific ResourceAttributes.
Users should not call this method directly; rather, they should run Resource.run or Resource.analyze.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
The resource that is being analyzed |
required |
config |
Optional config for analyzing. If an implementation provides a default, this default will always be used when config would otherwise be None. Note that a copy of the default config will be passed, so the default config values cannot be modified persistently by a component run. |
None |
Returns:
Type | Description |
---|---|
ElfDynamicEntry |
The analysis results |
Source code in ofrak/core/elf/analyzer.py
async def analyze(self, resource: Resource, config=None) -> ElfDynamicEntry:
deserializer = await _create_deserializer(resource)
return self.deserialize(deserializer)
ElfHeaderAttributesAnalyzer (Analyzer)
Deserialize the ElfHeader, which contains all of the ELF header fields except the first 7. The first 7 fields are deserialized as part of the ElfBasicHeader.
analyze(self, resource, config=None)
async
Analyze a resource for to extract specific ResourceAttributes.
Users should not call this method directly; rather, they should run Resource.run or Resource.analyze.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
The resource that is being analyzed |
required |
config |
Optional config for analyzing. If an implementation provides a default, this default will always be used when config would otherwise be None. Note that a copy of the default config will be passed, so the default config values cannot be modified persistently by a component run. |
None |
Returns:
Type | Description |
---|---|
ElfHeader |
The analysis results |
Source code in ofrak/core/elf/analyzer.py
async def analyze(self, resource: Resource, config=None) -> ElfHeader:
deserializer = await _create_deserializer(resource)
return self.deserialize(deserializer)
ElfPointerAnalyzer (Analyzer)
analyze(self, resource, config=None)
async
Analyze a resource for to extract specific ResourceAttributes.
Users should not call this method directly; rather, they should run Resource.run or Resource.analyze.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
The resource that is being analyzed |
required |
config |
Optional config for analyzing. If an implementation provides a default, this default will always be used when config would otherwise be None. Note that a copy of the default config will be passed, so the default config values cannot be modified persistently by a component run. |
None |
Returns:
Type | Description |
---|---|
ElfVirtualAddress |
The analysis results |
Source code in ofrak/core/elf/analyzer.py
async def analyze(self, resource: Resource, config=None) -> ElfVirtualAddress:
deserializer = await _create_deserializer(resource)
return self.deserialize(deserializer)
ElfProgramAttributesAnalyzer (Analyzer)
Analyze the ProgramAttributes
of an ELF, which are part of the information stored in the ELF
header.
analyze(self, resource, config=None)
async
Analyze a resource for to extract specific ResourceAttributes.
Users should not call this method directly; rather, they should run Resource.run or Resource.analyze.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
The resource that is being analyzed |
required |
config |
Optional[ofrak.model.component_model.ComponentConfig] |
Optional config for analyzing. If an implementation provides a default, this default will always be used when config would otherwise be None. Note that a copy of the default config will be passed, so the default config values cannot be modified persistently by a component run. |
None |
Returns:
Type | Description |
---|---|
ProgramAttributes |
The analysis results |
Source code in ofrak/core/elf/analyzer.py
async def analyze(
self, resource: Resource, config: Optional[ComponentConfig] = None
) -> ProgramAttributes:
elf_header = await resource.get_only_descendant_as_view(
ElfHeader, r_filter=ResourceFilter.with_tags(ElfHeader)
)
elf_basic_header = await resource.get_only_descendant_as_view(
ElfBasicHeader, r_filter=ResourceFilter.with_tags(ElfBasicHeader)
)
return ProgramAttributes(
elf_header.get_isa(),
None,
elf_basic_header.get_bitwidth(),
elf_basic_header.get_endianness(),
None,
)
ElfProgramHeaderAttributesAnalyzer (Analyzer)
Deserialize an ElfProgramHeader.
analyze(self, resource, config=None)
async
Analyze a resource for to extract specific ResourceAttributes.
Users should not call this method directly; rather, they should run Resource.run or Resource.analyze.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
The resource that is being analyzed |
required |
config |
Optional config for analyzing. If an implementation provides a default, this default will always be used when config would otherwise be None. Note that a copy of the default config will be passed, so the default config values cannot be modified persistently by a component run. |
None |
Returns:
Type | Description |
---|---|
ElfProgramHeader |
The analysis results |
Source code in ofrak/core/elf/analyzer.py
async def analyze(self, resource: Resource, config=None) -> ElfProgramHeader:
segment_structure = await resource.view_as(ElfSegmentStructure)
deserializer = await _create_deserializer(resource)
return self.deserialize(deserializer, segment_structure.segment_index)
ElfRelaAnalyzer (Analyzer)
Deserialize an ElfRelaEntry, an entry in a rela.* table.
http://sourceware.org/git/?p=glibc.git;a=blob_plain;f=elf/elf.h
analyze(self, resource, config=None)
async
Analyze a resource for to extract specific ResourceAttributes.
Users should not call this method directly; rather, they should run Resource.run or Resource.analyze.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
The resource that is being analyzed |
required |
config |
Optional config for analyzing. If an implementation provides a default, this default will always be used when config would otherwise be None. Note that a copy of the default config will be passed, so the default config values cannot be modified persistently by a component run. |
None |
Returns:
Type | Description |
---|---|
ElfRelaEntry |
The analysis results |
Source code in ofrak/core/elf/analyzer.py
async def analyze(self, resource: Resource, config=None) -> ElfRelaEntry:
deserializer = await _create_deserializer(resource)
return self.deserialize(deserializer)
ElfSectionHeaderAttributesAnalyzer (Analyzer)
Deserialize an ElfSectionHeader.
analyze(self, resource, config=None)
async
Analyze a resource for to extract specific ResourceAttributes.
Users should not call this method directly; rather, they should run Resource.run or Resource.analyze.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
The resource that is being analyzed |
required |
config |
Optional config for analyzing. If an implementation provides a default, this default will always be used when config would otherwise be None. Note that a copy of the default config will be passed, so the default config values cannot be modified persistently by a component run. |
None |
Returns:
Type | Description |
---|---|
ElfSectionHeader |
The analysis results |
Source code in ofrak/core/elf/analyzer.py
async def analyze(self, resource: Resource, config=None) -> ElfSectionHeader:
section_structure = await resource.view_as(ElfSectionStructure)
deserializer = await _create_deserializer(resource)
return self.deserialize(deserializer, section_structure.section_index)
ElfSectionMemoryRegionAnalyzer (Analyzer)
Get the in-memory address and size of an ELF section. These are stored in the corresponding ELF section header.
analyze(self, resource, config=None)
async
Analyze a resource for to extract specific ResourceAttributes.
Users should not call this method directly; rather, they should run Resource.run or Resource.analyze.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
The resource that is being analyzed |
required |
config |
Optional config for analyzing. If an implementation provides a default, this default will always be used when config would otherwise be None. Note that a copy of the default config will be passed, so the default config values cannot be modified persistently by a component run. |
None |
Returns:
Type | Description |
---|---|
MemoryRegion |
The analysis results |
Source code in ofrak/core/elf/analyzer.py
async def analyze(self, resource: Resource, config=None) -> MemoryRegion:
section = await resource.view_as(ElfSectionStructure)
section_header = await section.get_header()
return MemoryRegion(
virtual_address=section_header.sh_addr,
size=section_header.sh_size,
)
ElfSectionNameAnalyzer (Analyzer)
Get the name of an ELF section. ELF section names are stored as null-terminated strings in
dedicated string section, and each ELF section header's sh_name
field is an offset in this
section.
analyze(self, resource, config=None)
async
Analyze a resource for to extract specific ResourceAttributes.
Users should not call this method directly; rather, they should run Resource.run or Resource.analyze.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
The resource that is being analyzed |
required |
config |
Optional config for analyzing. If an implementation provides a default, this default will always be used when config would otherwise be None. Note that a copy of the default config will be passed, so the default config values cannot be modified persistently by a component run. |
None |
Returns:
Type | Description |
---|---|
AttributesType[NamedProgramSection] |
The analysis results |
Source code in ofrak/core/elf/analyzer.py
async def analyze(self, resource: Resource, config=None) -> AttributesType[NamedProgramSection]:
section = await resource.view_as(ElfSectionStructure)
section_header = await section.get_header()
elf_r = await section.get_elf()
string_section = await elf_r.get_section_name_string_section()
try:
((_, raw_section_name),) = await string_section.resource.search_data(
SECTION_NAME_PATTERN, start=section_header.sh_name, max_matches=1
)
section_name = raw_section_name.rstrip(b"\x00").decode("ascii")
except ValueError as e:
LOGGER.info("String section is empty! Using '<no-strings>' as section name")
section_name = "<no-strings>" # This is what readelf returns in this situation
return AttributesType[NamedProgramSection](
name=section_name,
)
ElfSegmentAnalyzer (Analyzer)
Analyze an ElfSegment.
analyze(self, resource, config=None)
async
Analyze a resource for to extract specific ResourceAttributes.
Users should not call this method directly; rather, they should run Resource.run or Resource.analyze.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
The resource that is being analyzed |
required |
config |
Optional config for analyzing. If an implementation provides a default, this default will always be used when config would otherwise be None. Note that a copy of the default config will be passed, so the default config values cannot be modified persistently by a component run. |
None |
Returns:
Type | Description |
---|---|
ElfSegment |
The analysis results |
Source code in ofrak/core/elf/analyzer.py
async def analyze(self, resource: Resource, config=None) -> ElfSegment:
segment = await resource.view_as(ElfSegmentStructure)
segment_header = await segment.get_header()
return ElfSegment(
segment_index=segment_header.segment_index,
virtual_address=segment_header.p_vaddr,
size=segment_header.p_memsz,
)
ElfSymbolAttributesAnalyzer (Analyzer)
Deserialize an ElfSymbol, an entry in the ELF symbol table.
analyze(self, resource, config=None)
async
Analyze a resource for to extract specific ResourceAttributes.
Users should not call this method directly; rather, they should run Resource.run or Resource.analyze.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
The resource that is being analyzed |
required |
config |
Optional config for analyzing. If an implementation provides a default, this default will always be used when config would otherwise be None. Note that a copy of the default config will be passed, so the default config values cannot be modified persistently by a component run. |
None |
Returns:
Type | Description |
---|---|
ElfSymbol |
The analysis results |
Source code in ofrak/core/elf/analyzer.py
async def analyze(self, resource: Resource, config=None) -> ElfSymbol:
symbol_structure = await resource.view_as(ElfSymbolStructure)
deserializer = await _create_deserializer(resource)
return self.deserialize(deserializer, symbol_structure.symbol_index)
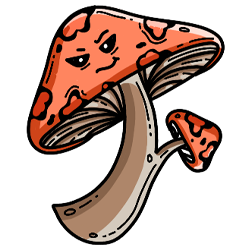