program.py
ofrak.core.program
Program (LinkableBinary)
Generic representation for a binary program with functions, code and memory regions, and ProgramAttributes.
get_code_regions(self)
async
Return code regions associated with the resource
Returns:
Type | Description |
---|---|
Iterable[ofrak.core.code_region.CodeRegion] |
Iterable of CodeRegions |
Source code in ofrak/core/program.py
async def get_code_regions(self) -> Iterable[CodeRegion]:
"""
Return code regions associated with the resource
:return: Iterable of CodeRegions
"""
return await self.resource.get_children_as_view(
CodeRegion, r_filter=ResourceFilter.with_tags(CodeRegion)
)
get_code_region_for_vaddr(self, vaddr)
async
Return the code region in this program containing vaddr.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
vaddr |
int |
Virtual address |
required |
Returns:
Type | Description |
---|---|
Optional[ofrak.core.code_region.CodeRegion] |
Code region containing the input vaddr |
Exceptions:
Type | Description |
---|---|
NotFoundError |
If vaddr is not in any code region |
Source code in ofrak/core/program.py
async def get_code_region_for_vaddr(self, vaddr: int) -> Optional[CodeRegion]:
"""
Return the code region in this program containing vaddr.
:param vaddr: Virtual address
:raises NotFoundError: If vaddr is not in any code region
:return: Code region containing the input vaddr
"""
code_regions = await self.get_code_regions()
for cr_view in code_regions:
code_region_vaddr_range = Range(
cr_view.virtual_address,
cr_view.virtual_address + cr_view.size,
)
if vaddr in code_region_vaddr_range:
return cr_view
raise NotFoundError
get_memory_region_for_vaddr(self, vaddr)
async
Return the largest memory region containing vaddr.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
vaddr |
int |
Virtual address |
required |
Returns:
Type | Description |
---|---|
Optional[ofrak.core.memory_region.MemoryRegion] |
The most general (largest) region of memory containing the input vaddr, if such a memory region exists |
Exceptions:
Type | Description |
---|---|
NotFoundError |
If vaddr is not in any memory region |
Source code in ofrak/core/program.py
async def get_memory_region_for_vaddr(self, vaddr: int) -> Optional[MemoryRegion]:
"""
Return the largest [memory region][ofrak.core.memory_region.MemoryRegion] containing vaddr.
:param vaddr: Virtual address
:raises NotFoundError: If vaddr is not in any memory region
:return: The most general (largest) region of memory containing the input vaddr,
if such a memory region exists
"""
# we're looking for the largest (most general) memory region containing this vaddr
mem_regions = await self.resource.get_descendants_as_view(
MemoryRegion,
r_filter=ResourceFilter.with_tags(MemoryRegion),
r_sort=ResourceSort(
attribute=MemoryRegion.Size,
direction=ResourceSortDirection.DESCENDANT,
),
)
return MemoryRegion.get_mem_region_with_vaddr_from_sorted(vaddr, mem_regions)
ReferencedDataAnalyzer (Analyzer, ABC)
analyze(self, resource, config=None)
async
Analyze a resource for to extract specific ResourceAttributes.
Users should not call this method directly; rather, they should run Resource.run or Resource.analyze.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
The resource that is being analyzed |
required |
config |
Optional config for analyzing. If an implementation provides a default, this default will always be used when config would otherwise be None. Note that a copy of the default config will be passed, so the default config values cannot be modified persistently by a component run. |
None |
Returns:
Type | Description |
---|---|
Tuple[ofrak.core.data.ReferencedDataAttributes] |
The analysis results |
Source code in ofrak/core/program.py
@abstractmethod
async def analyze(self, resource: Resource, config=None) -> Tuple[ReferencedDataAttributes]:
raise NotImplementedError()
ReferencedStringsAnalyzer (Analyzer, ABC)
analyze(self, resource, config=None)
async
Analyze a resource for to extract specific ResourceAttributes.
Users should not call this method directly; rather, they should run Resource.run or Resource.analyze.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
The resource that is being analyzed |
required |
config |
Optional config for analyzing. If an implementation provides a default, this default will always be used when config would otherwise be None. Note that a copy of the default config will be passed, so the default config values cannot be modified persistently by a component run. |
None |
Returns:
Type | Description |
---|---|
Tuple[ofrak.core.data.ReferencedStringsAttributes] |
The analysis results |
Source code in ofrak/core/program.py
@abstractmethod
async def analyze(self, resource: Resource, config=None) -> Tuple[ReferencedStringsAttributes]:
raise NotImplementedError()
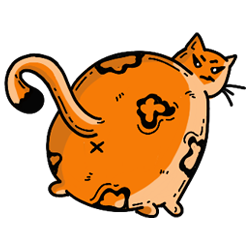