id_service_sequential.py
ofrak.service.id_service_sequential
SequentialIDService (IDServiceInterface)
An ID service implementation that generates sequencial ids to aid debugging.
This ID service is intended to be used for debugging purposes only, since it relies on its internal state to generate ids.
generate_id(self)
Generate a unique ID.
This method guarantees that no two IDs it returns will be identical for the lifetime of
the instantiated IDServiceInterface
.
Returns:
Type | Description |
---|---|
bytes |
The unique ID that was generated. |
Source code in ofrak/service/id_service_sequential.py
def generate_id(self) -> bytes:
_id = self._ids[self._scope]
self._ids[self._scope] += 1
return struct.pack(">I", _id)
generate_id_from_base(base_id, key)
staticmethod
Generate an ID based on a base ID and key.
This method returns the same ID each time it is called with the exact same parameters. It should therefore be used with caution: the callee needs to know that the parameters passed to this method are unique.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
base_id |
bytes |
An ID used to derive the generated ID. This ID can be assumed to have been generated by the |
required |
key |
str |
A key used to guarantee that the generated ID is unique. The callee is responsible for ensuring that this key is unique. |
required |
Returns:
Type | Description |
---|---|
bytes |
The ID that was generated. |
Source code in ofrak/service/id_service_sequential.py
@staticmethod
def generate_id_from_base(base_id: bytes, key: str) -> bytes:
return b"-".join((base_id, bytes(key, "utf-8")))
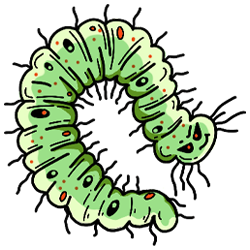