gnu.py
ofrak_patch_maker.binary_parser.gnu
GNU_ELF_Parser (AbstractBinaryFileParser)
parse_symbols(self, output)
Use objdump
with the --syms
flag to get info on all defined symbols in a file. Parses
columns based on: https://stackoverflow.com/a/16471895/16690095.
Source code in ofrak_patch_maker/binary_parser/gnu.py
def parse_symbols(self, output: str) -> Dict[str, Tuple[int, LinkableSymbolType]]:
"""
Use `objdump` with the `--syms` flag to get info on all defined symbols in a file. Parses
columns based on: <https://stackoverflow.com/a/16471895/16690095>.
"""
result = {}
symbols = self._get_all_symbols(output)
for sym_name, sym_vaddr, sym_section, sym_type in symbols:
if sym_section != "*UND*" and "w" not in sym_type:
if "F" in sym_type:
result[sym_name] = (sym_vaddr, LinkableSymbolType.FUNC)
else:
# TODO: handle data symbols and distinguish between RO and RW symbols with section info
result[sym_name] = (sym_vaddr, LinkableSymbolType.UNDEF)
return result
parse_sections(self, output)
Uses objdump
with the --section-headers
flag to get info on all symbols in a file.
Parses the returned columns.
Source code in ofrak_patch_maker/binary_parser/gnu.py
def parse_sections(self, output: str) -> Tuple[Segment, ...]:
"""
Uses `objdump` with the `--section-headers` flag to get info on all symbols in a file.
Parses the returned columns.
"""
segments = []
for section_data in self._re_section_prog.finditer(output):
# Default permissions are RW, then -R/+X as appropriate
permissions = MemoryPermissions.RW
if "READONLY" in section_data.group("flags"):
permissions = permissions - MemoryPermissions.W
if "CODE" in section_data.group("flags"):
permissions = permissions + MemoryPermissions.X
# TODO: Figure out how to infer this.
is_entry = False
seg = Segment(
segment_name=section_data.group("name"),
vm_address=int(section_data.group("vma"), 16),
offset=int(section_data.group("offset"), 16),
is_entry=is_entry,
length=int(section_data.group("size"), 16),
access_perms=permissions,
)
segments.append(seg)
return tuple(segments)
parse_relocations(self, output)
Use objdump
with the --syms
flag to get info on all undefined symbols in a file. Parses
columns based on: https://stackoverflow.com/a/16471895/16690095.
Source code in ofrak_patch_maker/binary_parser/gnu.py
def parse_relocations(self, output: str) -> Dict[str, Tuple[int, LinkableSymbolType]]:
"""
Use `objdump` with the `--syms` flag to get info on all undefined symbols in a file. Parses
columns based on: <https://stackoverflow.com/a/16471895/16690095>.
"""
result = {}
symbols = self._get_all_symbols(output)
for sym_name, sym_vaddr, sym_section, sym_type in symbols:
if sym_section == "*UND*" or "w" in sym_type:
result[sym_name] = (sym_vaddr, LinkableSymbolType.UNDEF)
return result
GNU_V10_ELF_Parser (GNU_ELF_Parser)
parse_symbols(self, tool_output)
Use objdump
with the --syms
flag to get info on all defined symbols in a file. Parses
columns based on: https://stackoverflow.com/a/16471895/16690095.
Source code in ofrak_patch_maker/binary_parser/gnu.py
def parse_symbols(self, tool_output: str) -> Dict[str, Tuple[int, LinkableSymbolType]]:
symbols = {}
lines = tool_output.split("\n")
for l in lines:
tokens = l.split()
if "O" in tokens or "F" in tokens:
symbols.update({tokens[-1]: (int(tokens[0], 16), LinkableSymbolType.FUNC)})
return symbols
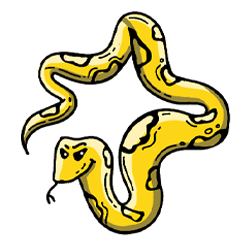