resource_indexed_attribute_serializer.py
ofrak.service.serialization.serializers.resource_indexed_attribute_serializer
ResourceIndexedAttributeSerializer (SerializerInterface)
Serialize and deserialize ResourceIndexedAttribute
and ResourceIndexedAttribute[T]
into PJSONType
.
A ResourceIndexedAttributes instance can contain a Callable, which is hard to serialize. However, we can recover this ResourceIndexedAttributes instance if we know its attributes_owner and its name, both being easy to serialize.
obj_to_pjson(self, obj, type_hint)
Serialize the object to PJSON. Note that the generic self._service.to_pjson
can be used here.
Source code in ofrak/service/serialization/serializers/resource_indexed_attribute_serializer.py
def obj_to_pjson(self, obj: Any, type_hint: Any) -> Dict[str, PJSONType]:
if obj.attributes_owner is None:
raise TypeError(f"Cannot serialize {obj.__name__} because its owner has not been set.")
else:
return {
"attributes_owner": self._service.to_pjson(
obj.attributes_owner, Type[ResourceAttributes]
),
"name": obj.index_name,
}
pjson_to_obj(self, pjson_obj, type_hint)
Deserialize PJSON into the object. Note that the generic self._service.from_pjson
can be used here.
Source code in ofrak/service/serialization/serializers/resource_indexed_attribute_serializer.py
def pjson_to_obj(self, pjson_obj: Dict[str, PJSONType], type_hint: Any) -> "ResourceIndexedAttribute": # type: ignore
attributes_owner = self._service.from_pjson(
pjson_obj["attributes_owner"], Type[ResourceAttributes]
)
name = self._service.from_pjson(pjson_obj["name"], str)
return getattr(attributes_owner, name)
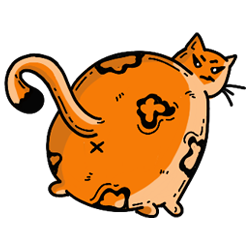