job_service_i.py
ofrak.service.job_service_i
JobServiceInterface (AbstractOfrakService)
Job service interface.
run_component(self, request, job_context=None)
async
Run a single component for a job.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
request |
JobComponentRequest |
required | |
job_context |
Optional[ofrak.model.job_model.JobRunContext] |
Context of the job to run the component in. |
None |
Returns:
Type | Description |
---|---|
ComponentRunResult |
A data structure describing the component run and resources modified/created/deleted. |
Source code in ofrak/service/job_service_i.py
@abstractmethod
async def run_component(
self,
request: JobComponentRequest,
job_context: Optional[JobRunContext] = None,
) -> ComponentRunResult:
"""
Run a single component for a job.
:param request:
:param job_context: Context of the job to run the component in.
:return: A data structure describing the component run and resources
modified/created/deleted.
"""
run_analyzer_by_attribute(self, request, job_context=None)
async
Choose one or more Analyzer components to analyze the requested attributes on the given resource.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
request |
JobAnalyzerRequest |
Data structure containing the ID of the job to run the components in, the ID of the resource to run components on, which attributes the Analyzers should output, and the tags of the target resource. |
required |
job_context |
Optional[ofrak.model.job_model.JobRunContext] |
Context of the job to run the component in. |
None |
Returns:
Type | Description |
---|---|
ComponentRunResult |
A data structure describing the component(s) run and resources modified/created/deleted. |
Exceptions:
Type | Description |
---|---|
NotFoundError |
If no Analyzers can be found targeting the specified tags and outputting the specified attributes. |
Source code in ofrak/service/job_service_i.py
@abstractmethod
async def run_analyzer_by_attribute(
self,
request: JobAnalyzerRequest,
job_context: Optional[JobRunContext] = None,
) -> ComponentRunResult:
"""
Choose one or more Analyzer components to analyze the requested attributes on the given
resource.
:param request: Data structure containing the ID of the job to run the components in,
the ID of the resource to run components on, which attributes the Analyzers should
output, and the tags of the target resource.
:param job_context: Context of the job to run the component in.
:return: A data structure describing the component(s) run and resources
modified/created/deleted.
:raises NotFoundError: If no Analyzers can be found targeting the specified tags and
outputting the specified attributes.
"""
run_components(self, request)
async
Automatically select one or more components to run on a resource. The components must match the provided component filters and target at least one of the tags of the resource.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
request |
JobMultiComponentRequest |
Data structure containing the ID of the job to run the components in, the ID of the resource to run components on, and filters for the components to run. |
required |
Returns:
Type | Description |
---|---|
ComponentRunResult |
A data structure describing the components run and resources modified/created/deleted. |
Exceptions:
Type | Description |
---|---|
ComponentAutoRunFailure |
if one of the automatically chosen components raises an error while running. |
NoMatchingComponentException |
if no components match the filters for the resource. |
Source code in ofrak/service/job_service_i.py
@abstractmethod
async def run_components(
self,
request: JobMultiComponentRequest,
) -> ComponentRunResult:
"""
Automatically select one or more components to run on a resource. The components must
match the provided component filters and target at least one of the tags of the resource.
:param request: Data structure containing the ID of the job to run the components in,
the ID of the resource to run components on, and filters for the components to
run.
:return: A data structure describing the components run and resources
modified/created/deleted.
:raises ComponentAutoRunFailure: if one of the automatically chosen components raises an
error while running.
:raises NoMatchingComponentException: if no components match the filters for the resource.
"""
run_components_recursively(self, request)
async
Start from a resource and run components on it and then on any resources which have tags added as a result of that initial run, then run components on any resources with new tags from those subsequent runs, until an iteration of component runs results in no new tags being added. The component(s) run on each resource are chosen according to the provided filters and which tags were added to that resource in the previous iteration. That is, the filters are applied to the set of resource which target those new tags.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
request |
JobMultiComponentRequest |
Data structure containing the ID of the job to run the components in, the ID of the resource to start running recursively from, and filters for the components to run. |
required |
Returns:
Type | Description |
---|---|
ComponentRunResult |
A data structure describing the components run and resources modified/created/deleted. |
Exceptions:
Type | Description |
---|---|
ComponentAutoRunFailure |
if one of the automatically chosen components raises an error while running. |
Source code in ofrak/service/job_service_i.py
@abstractmethod
async def run_components_recursively(
self,
request: JobMultiComponentRequest,
) -> ComponentRunResult:
"""
Start from a resource and run components on it and then on any resources which have tags
added as a result of that initial run, then run components on any resources with new tags
from those subsequent runs, until an iteration of component runs results in no new tags
being added. The component(s) run on each resource are chosen according to the provided
filters and which tags were added to that resource in the previous iteration. That is,
the filters are applied to the set of resource which target those new tags.
:param request: Data structure containing the ID of the job to run the components in,
the ID of the resource to start running recursively from, and filters for the components to
run.
:return: A data structure describing the components run and resources
modified/created/deleted.
:raises ComponentAutoRunFailure: if one of the automatically chosen components raises an
error while running.
"""
pack_recursively(self, job_id, resource_id)
async
Call Packer components on the deepest descendants of a resource (the root of this search), then Packers on the next level up, etc. until the search root resource.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
job_id |
bytes |
Job to run the component in. |
required |
resource_id |
bytes |
ID of the search root resource. |
required |
Returns:
Type | Description |
---|---|
ComponentRunResult |
A data structure describing the components run and resources modified/created/deleted. |
Source code in ofrak/service/job_service_i.py
@abstractmethod
async def pack_recursively(
self,
job_id: bytes,
resource_id: bytes,
) -> ComponentRunResult:
"""
Call Packer components on the deepest descendants of a resource (the root of this search),
then Packers on the next level up, etc. until the search root resource.
:param job_id: Job to run the component in.
:param resource_id: ID of the search root resource.
:return: A data structure describing the components run and resources
modified/created/deleted.
"""
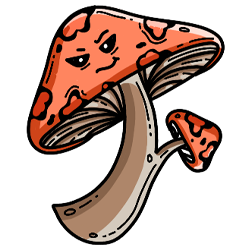