basic_block.py
ofrak.core.basic_block
BasicBlock (MemoryRegion)
dataclass
A collection of instructions that constitute a single block of code with one entry point and one exit point.
Attributes:
Name | Type | Description |
---|---|---|
virtual_address |
int |
the virtual address corresponding ot the start of the basic block |
size |
int |
the basic block's size (the difference between the virtual address immediately after the block's last instruction and the block's virtual address) |
mode |
InstructionSetMode |
the instruction set mode of the basic block |
is_exit_point |
bool |
true if the basic block is the exit point of a function; if so, then exit_vaddr is None |
exit_vaddr |
Optional[int] |
the virtual address of the next basic block (if the current basic block is not an exit point) |
get_instructions(self)
async
Get the basic block's instructions.
Returns:
Type | Description |
---|---|
Iterable[ofrak.core.instruction.Instruction] |
the instructions in the basic block |
Source code in ofrak/core/basic_block.py
async def get_instructions(self) -> Iterable[Instruction]:
"""
Get the basic block's [instructions][ofrak.core.instruction.Instruction].
:return: the instructions in the basic block
"""
return await self.resource.get_descendants_as_view(
Instruction,
r_filter=ResourceFilter.with_tags(Instruction),
r_sort=ResourceSort(Addressable.VirtualAddress, ResourceSortDirection.ASCENDANT),
)
get_assembly(self)
async
Get the basic block's instructions as an assembly string.
Returns:
Type | Description |
---|---|
str |
the basic block's assembly |
Source code in ofrak/core/basic_block.py
async def get_assembly(self) -> str:
"""
Get the basic block's instructions as an assembly string.
:return: the basic block's assembly
"""
instructions = await self.get_instructions()
return "\n".join([i.get_assembly() for i in instructions])
BasicBlockAnalyzer (Analyzer, ABC)
Analyze a basic block and extract its virtual address, size, mode, whether it is an exit point, and exit_vaddr.
analyze(self, resource, config=None)
async
Analyze a basic block and extract its virtual address, size, mode, whether it is an exit point, and exit_vaddr.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
the basic block resource |
required |
config |
None |
Returns:
Type | Description |
---|---|
BasicBlock |
the analyzed basic block |
Source code in ofrak/core/basic_block.py
@abstractmethod
async def analyze(self, resource: Resource, config=None) -> BasicBlock:
"""
Analyze a basic block and extract its virtual address, size, mode, whether it is an
exit point, and exit_vaddr.
:param resource: the basic block resource
:param config:
:return: the analyzed basic block
"""
raise NotImplementedError()
BasicBlockUnpacker (Unpacker, ABC)
Unpack a basic block into instructions.
unpack(self, resource, config=None)
async
Unpack a basic block into its corresponding instructions.
Source code in ofrak/core/basic_block.py
@abstractmethod
async def unpack(self, resource: Resource, config=None):
"""
Unpack a basic block into its corresponding instructions.
"""
raise NotImplementedError()
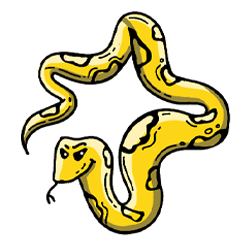