ghidra_decompilation_analyzer.py
ofrak_ghidra.components.ghidra_decompilation_analyzer
GhidraDecompilationAnalyzer (DecompilationAnalyzer, OfrakGhidraMixin)
analyze(self, resource, config)
async
Analyze a complex block resource and extract its decompilation as a string.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
the complex block resource |
required |
config |
None |
required |
Returns:
Type | Description |
---|---|
DecompilationAnalysis |
the decompilation |
Source code in ofrak_ghidra/components/ghidra_decompilation_analyzer.py
async def analyze(self, resource: Resource, config: None) -> DecompilationAnalysis:
# Run / fetch ghidra analyzer
complex_block = await resource.view_as(ComplexBlock)
result = {}
try:
result = await self.get_decompilation_script.call_script(
resource, complex_block.virtual_address
)
except JSONDecodeError as e:
return DecompilationAnalysis(str(e))
if "decomp" in result:
decomp = (
result["decomp"]
.replace("<quote>", "'")
.replace("<dquote>", '"')
.replace("<nl>", "\n")
.replace("<cr>", "\r")
.replace("<tab>", "\t")
.replace("<zero>", "\\0")
)
else:
decomp = "No Decompilation available"
decomp_escaped = escape_strings(decomp)
return DecompilationAnalysis(decomp_escaped)
escape_strings(s)
Escape newlines in strings (enclosed by double quotes) and characters (enclosed by single quotes).
If this isn't done, there will be a linebreak in the decompilation string/view in the middle of a C string or char.
Source code in ofrak_ghidra/components/ghidra_decompilation_analyzer.py
def escape_strings(s: str) -> str:
"""
Escape newlines in strings (enclosed by double quotes) and
characters (enclosed by single quotes).
If this isn't done, there will be a linebreak in the decompilation
string/view in the middle of a C string or char.
"""
s_escaped = ""
while '"' in s or "'" in s:
(s, escaped_string) = take_delimited(s, '"')
s_escaped += escaped_string
(s, escaped_char) = take_delimited(s, "'")
s_escaped += escaped_char
s_escaped += s
return s_escaped
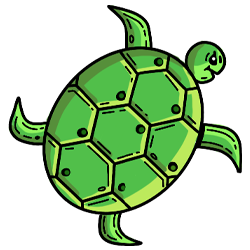