lzma.py
ofrak.core.lzma
LzmaData (GenericBinary)
An lzma binary blob.
LzmaPacker (Packer)
Pack data into a compressed LZMA | XZ file.
pack(self, resource, config=None)
async
Pack the given resource.
Users should not call this method directly; rather, they should run Resource.run or Resource.pack.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
required | |
config |
Optional config for packing. If an implementation provides a default, this default will always be used when config would otherwise be None. Note that a copy of the default config will be passed, so the default config values cannot be modified persistently by a component run. |
None |
Source code in ofrak/core/lzma.py
async def pack(self, resource: Resource, config=None):
lzma_format, tag = await self._get_lzma_format_and_tag(resource)
lzma_file: Union[XzData, LzmaData] = await resource.view_as(tag)
lzma_child = await lzma_file.get_child()
lzma_compressed = lzma.compress(await lzma_child.resource.get_data(), lzma_format)
original_size = await lzma_file.resource.get_data_length()
resource.queue_patch(Range(0, original_size), lzma_compressed)
LzmaUnpacker (Unpacker)
Unpack (decompress) an LZMA | XZ file.
unpack(self, resource, config=None)
async
Unpack the given resource.
Users should not call this method directly; rather, they should run Resource.run or Resource.unpack.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
The resource that is being unpacked |
required |
config |
Optional config for unpacking. If an implementation provides a default, this default will always be used when config would otherwise be None. Note that a copy of the default config will be passed, so the default config values cannot be modified persistently by a component run. |
None |
Source code in ofrak/core/lzma.py
async def unpack(self, resource: Resource, config=None):
file_data = BytesIO(await resource.get_data())
format = lzma.FORMAT_AUTO
if resource.has_tag(XzData):
format = lzma.FORMAT_XZ
elif resource.has_tag(LzmaData):
format = lzma.FORMAT_ALONE
lzma_entry_data = None
compressed_data = file_data.read()
try:
lzma_entry_data = lzma.decompress(compressed_data, format)
except lzma.LZMAError:
LOGGER.info("Initial LZMA decompression failed. Trying with null bytes stripped")
lzma_entry_data = lzma.decompress(compressed_data.rstrip(b"\x00"), format)
if lzma_entry_data is not None:
await resource.create_child(
tags=(GenericBinary,),
data=lzma_entry_data,
)
else:
raise lzma.LZMAError("Decompressed LZMA data is null")
XzData (GenericBinary)
An xz binary blob.
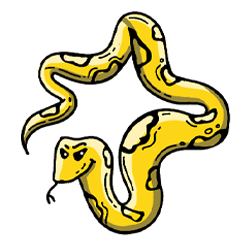