data.py
ofrak.core.data
DataWord (MemoryRegion)
dataclass
Addressable data word. Size depends on target system, and is extracted by analysis backend.
Attributes:
Name | Type | Description |
---|---|---|
virtual_address |
int |
virtual address of the memory region containing the data word |
size |
int |
size of the memory region containing the data word |
format_string |
str |
|
xrefs_to |
Tuple[int, ...] |
cross references to the data word |
ReferencedDataAttributes (ResourceAttributes)
dataclass
Data cross-references in a program. That is, all addresses which are known to reference data and all data addresses which are known to be referenced.
Attributes:
Name | Type | Description |
---|---|---|
referencing_addresses |
Tuple[int, ...] |
Addresses of all items which reference data in the program |
referenced_data |
Tuple[int, ...] |
All data which are referenced in the program |
references |
Tuple[Tuple[int, int], ...] |
Pairs of indexes in |
get_xrefs_to(self)
Create a dictionary version of the data ref information. The keys are data addresses, and the value for each of those is a tuple of all the addresses which reference that data address.
Returns:
Type | Description |
---|---|
Dict[int, Tuple[int, ...]] |
Dictionary mapping each data address to the addresses that reference it |
Source code in ofrak/core/data.py
def get_xrefs_to(self) -> Dict[int, Tuple[int, ...]]:
"""
Create a dictionary version of the data ref information. The keys are data addresses, and
the value for each of those is a tuple of all the addresses which reference that data
address.
:return: Dictionary mapping each data address to the addresses that reference it
"""
data_vaddrs_to_referrees = defaultdict(list)
for ref_from_idx, ref_to_idx in self.references:
data_vaddrs_to_referrees[self.referenced_data[ref_to_idx]].append(
self.referencing_addresses[ref_from_idx]
)
return {
data_vaddr: tuple(addresses_refering_to_data_vaddr)
for data_vaddr, addresses_refering_to_data_vaddr in data_vaddrs_to_referrees.items()
}
get_xrefs_from(self)
Create a dictionary version of the data ref information. The keys are addresses, and the value for each of those is a tuple of all the data addresses are referenced from that address.
Returns:
Type | Description |
---|---|
Dictionary mapping each address to the addresses of the data that it references |
Source code in ofrak/core/data.py
def get_xrefs_from(self):
"""
Create a dictionary version of the data ref information. The keys are addresses, and
the value for each of those is a tuple of all the data addresses are referenced from that
address.
:return: Dictionary mapping each address to the addresses of the data that it references
"""
addresses_to_referenced_data = defaultdict(list)
for ref_from_idx, ref_to_idx in self.references:
addresses_to_referenced_data[self.referencing_addresses[ref_from_idx]].append(
self.referenced_data[ref_to_idx]
)
return {
vaddr: tuple(referenced_data_vaddrs)
for vaddr, referenced_data_vaddrs in addresses_to_referenced_data.items()
}
ReferencedStringsAttributes (ResourceAttributes)
dataclass
Strings referenced by functions, represented as ResourceAttributes.
Attributes:
Name | Type | Description |
---|---|---|
referencing_functions |
Tuple[int, ...] |
All functions which reference strings in the program |
referenced_strings |
Tuple[ofrak_type.range.Range, ...] |
All strings which are referenced in the program |
references |
Tuple[Tuple[int, int], ...] |
Edges from referencing funcs to referenced strings |
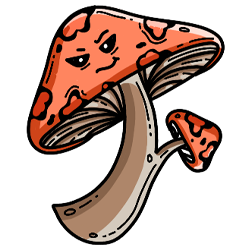