run_script_modifier.py
ofrak.core.run_script_modifier
RunScriptModifier (Modifier)
"Import" and run Python functions in the current OFRAK context. Useful for reusing quick helper functions and the like. Since this can be run through the GUI, it can be used to automate tasks which might be repetitive or impossible purely through the graphical interface.
modify(self, resource, config)
async
Modify the given resource.
Users should not call this method directly; rather, they should run Resource.run.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
required | |
config |
RunScriptModifierConfig |
Optional config for modification. If an implementation provides a default, this default will always be used when config would otherwise be None. Note that a copy of the default config will be passed, so the default config values cannot be modified persistently by a component run. |
required |
Source code in ofrak/core/run_script_modifier.py
async def modify(self, resource: Resource, config: RunScriptModifierConfig) -> None:
script_globals: Dict[str, Any] = dict()
script_locals: Dict[str, Any] = dict()
exec(config.code, script_globals, script_locals)
if config.function_name in script_globals:
script_main = script_globals[config.function_name]
elif config.function_name in script_locals:
script_main = script_locals[config.function_name]
else:
raise ValueError(f"No `{config.function_name}` function found in script!")
from ofrak.ofrak_context import get_current_ofrak_context
context = get_current_ofrak_context()
script_main.__globals__.update(script_globals)
script_main.__globals__.update(script_locals)
full_kwargs: Dict[str, Any] = {
"ofrak_context": context,
"root_resource": resource,
}
full_kwargs.update(config.extra_args)
await script_main(**full_kwargs)
RunScriptModifierConfig (ComponentConfig)
dataclass
Specification of some Python code to run in the current OFRAK context. Useful for reusing quick helper functions and the like.
Attributes:
Name | Type | Description |
---|---|---|
code |
str |
Python source code defining one or more function which take an OFRAKContext and Resource as arguments. |
function_name |
str |
Name of the function to run. |
extra_args |
Dict[str, Union[int, bool, str, float, bytes]] |
Extra arguments to pass to the function in key-value form. |
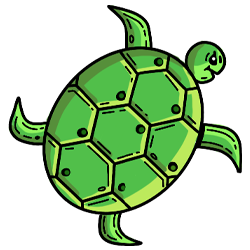