binwalk.py
ofrak_components.binwalk
BinwalkAnalyzer (Analyzer)
analyze(self, resource, config=None)
async
Analyze a resource for to extract specific ResourceAttributes.
Users should not call this method directly; rather, they should run Resource.run or Resource.analyze.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
resource |
Resource |
The resource that is being analyzed |
required |
config |
Optional config for analyzing. If an implementation provides a default, this default will always be used when config would otherwise be None. Note that a copy of the default config will be passed, so the default config values cannot be modified persistently by a component run. |
None |
Returns:
Type | Description |
---|---|
BinwalkAttributes |
The analysis results |
Source code in ofrak_components/binwalk.py
async def analyze(self, resource: Resource, config=None) -> BinwalkAttributes:
if not BINWALK_INSTALLED:
raise ComponentMissingDependencyError(self, BINWALK_TOOL)
with tempfile.NamedTemporaryFile() as temp_file:
data = await resource.get_data()
temp_file.write(data)
temp_file.flush()
# Should errors be handled the way they are in the `DataSummaryAnalyzer`? Likely to be
# overkill here.
offsets = await asyncio.get_running_loop().run_in_executor(
self.pool, _run_binwalk_on_file, temp_file.name
)
return BinwalkAttributes(offsets)
BinwalkAttributes (ResourceAttributes)
dataclass
BinwalkAttributes(offsets: Dict[int, str])
_BinwalkExternalTool (ComponentExternalTool)
private
__init__(self)
special
Initialize self. See help(type(self)) for accurate signature.
Source code in ofrak_components/binwalk.py
def __init__(self):
super().__init__(
"binwalk",
"https://github.com/ReFirmLabs/binwalk",
install_check_arg="",
)
is_tool_installed(self)
Check if a tool is installed by running it with the install_check_arg
.
This method runs <tool> <install_check_arg>
.
Returns:
Type | Description |
---|---|
bool |
True if the |
Source code in ofrak_components/binwalk.py
def is_tool_installed(self) -> bool:
return BINWALK_INSTALLED
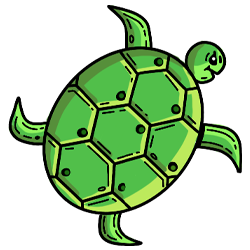